Ajax call
How to do manually using JavaScript
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="WebApplication1._Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script>
function loadXMLDoc()
{
var xmlhttp;
if (window.XMLHttpRequest)
{// code for IE7+, Firefox, Chrome, Opera, Safari
xmlhttp=new XMLHttpRequest();
}
else
{// code for IE6, IE5
xmlhttp=new ActiveXObject("Microsoft.XMLHTTP");
}
xmlhttp.onreadystatechange=function()
{
if (xmlhttp.readyState==4 && xmlhttp.status==200)
{
document.getElementById("myDiv").innerHTML=xmlhttp.responseText;
}
}
xmlhttp.open("GET","text.aspx",true);
xmlhttp.send();
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<div id="myDiv"><h2>Let AJAX change this text</h2></div>
<button type="button" onclick="loadXMLDoc()">Change Content</button>
</div>
</form>
</body>
</html>
Text.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="text.aspx.cs" Inherits="WebApplication1.text" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:DropDownList ID="me" runat="server">
<asp:ListItem>one</asp:ListItem>
<asp:ListItem>Two</asp:ListItem>
</asp:DropDownList>
</div>
</form>
</body>
</html>
.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace WebApplication1
{
public partial class text : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
Response.Write("Yahoo");
}
}
}
After Clicking 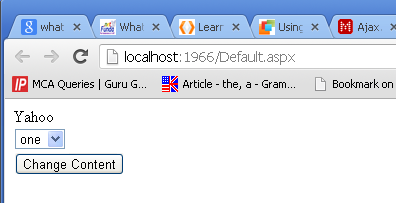
Ajax.net Example
Note: In this if btn1 is not registered with trigger through ControlID then clicking on btn1 will cause a postback. But by registering btn1 with trigger it uses ajax to call that function asynchronously.
Comments
Post a Comment